Three strategies for deploying container images to Amazon ECS using CDK
by Thorsten Höger, Cloud Automation Evangelist
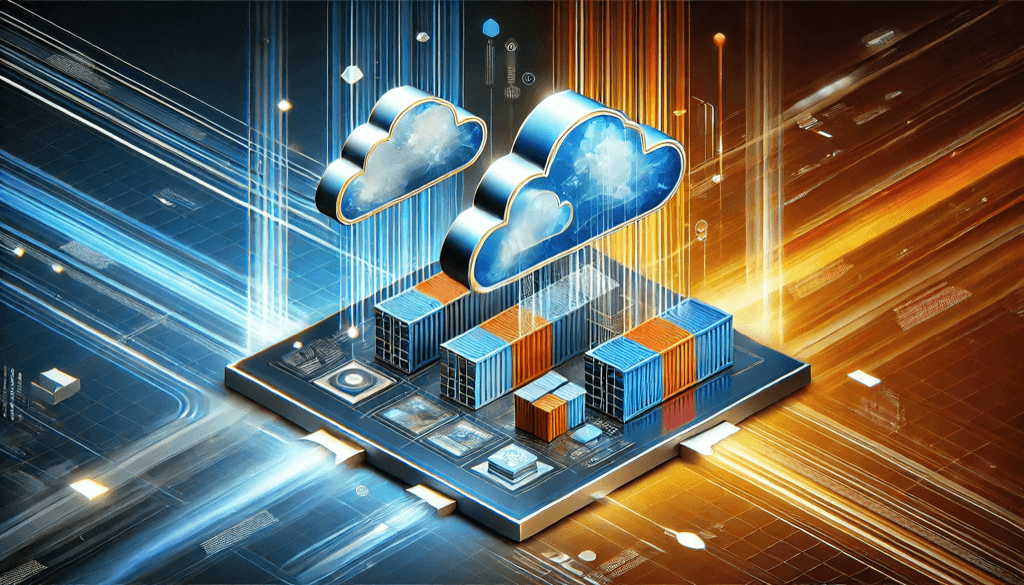
As containers continue to shape the cloud computing landscape, Amazon Elastic Container Service (ECS) remains a go-to choice for running containerized applications on AWS. However, deploying container images to ECS isn't a one-size-fits-all process. In this post, we'll explore three effective strategies for deploying container images to Amazon ECS, each tailored to different scenarios and offering distinct advantages.
Introduction
Amazon ECS simplifies the process of running, stopping, and managing Docker containers on a cluster of EC2 instances or managed instances using Fargate. As a fully managed container orchestration service, it allows you to focus on designing and building your applications rather than managing infrastructure.
When it comes to deploying container images to ECS, your chosen strategy can significantly impact your development workflow, deployment speed, and overall application lifecycle management. We'll look into three strategies:
- Using Pre-built Images
- Custom Images from Separate Projects
- Images Built Alongside CDK Code
Each of these strategies leverages the AWS Cloud Development Kit (CDK), enabling us to define our infrastructure as code and streamline our deployment processes.
Strategy 1: Using Pre-built Images
Overview and Use Cases
This strategy is ideal when working with existing images from public registries like Docker Hub, Artifactory, or public Amazon Elastic Container Registry (ECR). It's particularly useful when:
- You're using third-party images that don't require customization
- Your organization maintains a central repository of pre-approved images
- You're in the early stages of containerizing your application and want to start with a known, working image
Implementation Using AWS CDK
Let's walk through how to implement this strategy using AWS CDK:
- Ensure you have the AWS CDK installed and a CDK project set up.
- Import the necessary modules:
import { aws_ecs, aws_ec2 } from 'aws-cdk-lib';
- Create your ECS cluster:
const cluster = new aws_ecs.Cluster(this, 'MyCluster', {
vpc: new aws_ec2.Vpc(this, 'MyVpc')
});
- Define your task definition and use
aws_ecs.ContainerImage.fromRegistry()
to specify your pre-built image:
const taskDefinition = new aws_ecs.FargateTaskDefinition(this, 'TaskDef');
taskDefinition.addContainer('WebContainer', {
image: aws_ecs.ContainerImage.fromRegistry('nginx:latest'),
memoryLimitMiB: 512,
cpu: 256,
});
- Create your ECS service:
new aws_ecs.FargateService(this, 'MyService', {
cluster,
taskDefinition,
});
Pros and Cons
Pros:
- Quick and straightforward to implement
- No need to manage the image building process
- Easy to use well-maintained, public images
Cons:
- Limited control over the image contents
- Potential security risks if not using trusted sources
- May not be suitable for applications requiring custom configurations
Best Practices and Tips
- Always specify an exact image tag rather than using 'latest' to ensure reproducibility and updatability
- Regularly update your images to get the latest security patches
- Consider using ECR for private, pre-built images to benefit from integration with AWS services
- Consider SLAs and request limits from registries

Strategy 2: Custom Images from Separate Projects
Overview and Use Cases
This strategy is effective when you have custom images that are built in separate projects or CI/CD pipelines. It's particularly useful when:
- You have a dedicated team or process for building and maintaining Docker images
- Your images require complex build processes that are better managed separately
- You want to decouple image building from infrastructure deployment
Implementation Using AWS CDK
Here's how to implement this strategy:
- Ensure your custom image is built and pushed to a registry (e.g., ECR) in a separate process.
- In your CDK project, create a folder with a one-line Dockerfile that references your custom image: This way we make sure the image is referenced from our account's ECR and not dependent on the source repository
FROM 123456789012.dkr.ecr.us-west-2.amazonaws.com/my-app:1.2.3
- In your CDK stack, use
aws_cs.ContainerImage.fromAsset()
to reference this Dockerfile:
const taskDefinition = new aws_ecs.FargateTaskDefinition(this, 'TaskDef');
taskDefinition.addContainer('MyAppContainer', {
image: aws_ecs.ContainerImage.fromAsset('app'),
memoryLimitMiB: 512,
cpu: 256,
});
- Create your ECS service as before:
new aws_ecs.FargateService(this, 'MyService', {
cluster,
taskDefinition,
});
Pros and Cons
Pros:
- Separation of concerns between image building and infrastructure deployment
- Allows for complex, custom image building processes
- Enables use of specialized CI/CD pipelines for image building
Cons:
- Requires coordination between image building and infrastructure deployment processes
- May introduce complexity in version management
- Potential for misalignment between image and infrastructure versions
- More work to update the infrastructure when creating a new application version
Best Practices and Tips
- Use semantic versioning for your images to clearly communicate changes
- Implement a robust tagging strategy in your image building pipeline
- Implement a PR process to automatically create pull requests when new image versions are published

Strategy 3: Images Built Alongside CDK Code
Overview and Use Cases
This strategy involves building your Docker images directly within your CDK project. It's particularly useful when:
- You want tight integration between your application code and infrastructure definition
- Your images are relatively simple and don't require a complex build process
- You prefer a self-contained project where all components are managed together
Implementation Using AWS CDK
Here's how to implement this strategy:
- In your CDK project, create a folder with a Dockerfile for your application:
FROM node:20
WORKDIR /usr/src/app
COPY package*.json ./
RUN npm install
COPY . .
EXPOSE 8080
CMD [ "node", "server.js" ]
- In your CDK stack, use
aws_cs.ContainerImage.fromAsset()
to build and use this image:
import { aws_ecs } from 'aws-cdk-lib';
const taskDefinition = new aws_ecs.FargateTaskDefinition(this, 'TaskDef');
taskDefinition.addContainer('MyAppContainer', {
image: aws_ecs.ContainerImage.fromAsset('app'),
memoryLimitMiB: 512,
cpu: 256,
});
new ecs.FargateService(this, 'MyService', {
cluster,
taskDefinition,
});
In this setup, CDK will build the Docker image locally during synthesis and push it to ECR during deployment.
Pros and Cons
Pros:
- Tight integration between application code and infrastructure
- Self-contained project, easier to manage as a single unit
- Automatic handling of image building and pushing by CDK
Cons:
- May slow down CDK synthesis and deployment for complex images
- Less suitable for images that require very complex build processes
- Can make it harder to use specialized CI/CD tools for image building
Best Practices and Tips
- Keep your Dockerfile optimized for faster builds
- Use .dockerignore to exclude unnecessary files from the build context
- Consider using multi-stage builds to keep final images small

Comparison of Strategies
Here's a quick comparison to help you choose the right strategy for your needs:
Aspect | Pre-built Images | Custom Images from Separate Projects | Images Built Alongside CDK Code |
---|---|---|---|
Complexity | Low | Medium | Low to Medium |
Flexibility | Low | High | Medium |
Build Speed | N/A | Fast (pre-built) | Can be slow for complex images |
Integration with Infrastructure | Loose | Medium | Tight |
Suitable for Complex Images | No | Yes | Depends on complexity |
Version Control | Challenging | Good | Excellent |
Advanced Considerations
Security Best Practices
Regardless of the strategy you choose, consider these security best practices:
- Use minimal base images to reduce attack surface
- Implement least privilege principles in your task execution roles
- Regularly scan your images for vulnerabilities and include that in your pipeline
- Use AWS Secrets Manager to handle sensitive data
Optimizing for Performance and Cost
To optimize your ECS deployments:
- Use appropriate task sizes to avoid over-provisioning
- Implement scaling based on your application's needs
- Think about Spot instances for non-critical workloads
- Optimize your Docker images for size and startup time
Integration with CI/CD Pipelines
Each strategy can be integrated into CI/CD pipelines:
- For pre-built images, trigger automated pull requests and deployments when new versions are published
- For separate projects, use a pipeline to build images and another to deploy infrastructure
- For images built with CDK, include image building in your infrastructure deployment pipeline using
fromAsset
Conclusion
Choosing the right strategy for deploying container images to Amazon ECS is crucial for efficient and effective cloud operations. While each approach we've discussed has its merits, the third strategy - building images alongside CDK code - offers a compelling balance of integration and flexibility that's worth a closer look.
This approach shines in scenarios where you want to maintain a tight coupling between your application code and infrastructure definition. It's particularly powerful for teams embracing the "infrastructure as code" philosophy, as it allows for versioning both your application and infrastructure in a single repository. This can significantly streamline your CI/CD pipelines and make it easier to maintain consistency across environments.
However, remember that the best strategy is ultimately the one that aligns with your team's workflow and your application's specific requirements. Don't hesitate to mix and match these approaches as needed for different components of your system. The flexibility of containers and the AWS ecosystem allows for creative solutions to complex problems.
As container technologies continue to evolve, so too will these strategies. Stay engaged with the AWS community, keep an eye on new features and services, and be ready to adapt your approach as new best practices emerge.
Implementing these strategies effectively requires a deep understanding of both AWS services and container orchestration principles. If you find yourself needing guidance on optimizing your ECS deployments or architecting robust, scalable container solutions, consider reaching out to experienced professionals in the field.
As the author of this post, I've helped numerous organizations navigate the complexities of container deployment on AWS. If you're looking to elevate your container strategy or need assistance in implementing these approaches, feel free to connect. Together, we can design a container deployment strategy that not only meets your current needs but also positions you for future growth and innovation.
Remember, in the world of cloud computing, the right expertise can make all the difference.
Additional Resources
Happy containerizing!